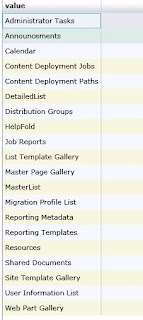
Finally and Simply,
I just figured out a mechanism to display SharePoint List using Silverlight. There could be many other simple ways, I can just say this is 1 of them. So how did I do it... keep reading...
I wanted to display all the List / Libraries for a given Site.
I created a solution with 2 projects. (I am just jumping into implementation and not adding any basic stuff such as installing Silverlight tools etc)
1. Silverlight project
a. Service References (Which would be our SharePointConnect project)
b. Page.xaml
c. ServiceReferences.ClientConfig
2. ASP.NET Web project
a. crossdomain.xml
b. ISPConnect.cs
c. SPConnect.svc
Lets start with first the SharePointConnect Project.
1. Add a WCF Service file (SPConnect in the above pic)
2. This would essentially add a Interface and the corresponding svc file. Also, add the reference to Microsoft.Sharepoint.dll
3. In the interface, you can add code as show below
using System;
using System.Collections.Generic;
using System.ServiceModel;
namespace SharePointConnect
{
[ServiceContract]
public interface ISPConnect
{
[OperationContract]
void DoWork();
[OperationContract]
ListGetSPListItems(string sUrl);
}
}
4. And the real implementation under SPConnect.svc.cs would be some thing like below..
using System;
using System.Collections.Generic;
using Microsoft.SharePoint;
namespace SharePointConnect
{
public class SPConnect : ISPConnect
{
public void DoWork()
{
}
public ListGetSPListItems(string Url)
{
ListsList = new List ();
using (SPSite WebApp = new SPSite(Url))
{
using (SPWeb site = WebApp.OpenWeb())
{
foreach (SPList currentList in site.Lists)
{
sList.Add(currentList.Title.ToString());
}
}
}
return sList;
}
}
}
5. By now if you open the web.config you must notice the below. Most importantly notice the basicHttpBinding mode (I would explain it in another post about basicHttpBinding).
<system.serviceModel>
<behaviors>
<serviceBehaviors>
<behavior name="SharePointConnect.SPConnectBehavior">
<serviceMetadata httpGetEnabled="true" />
<serviceDebug includeExceptionDetailInFaults="false" />
</behavior>
</serviceBehaviors>
</behaviors>
<services>
<service behaviorConfiguration="SharePointConnect.SPConnectBehavior"
name="SharePointConnect.SPConnect">
<endpoint address="" binding="basicHttpBinding" contract="SharePointConnect.ISPConnect">
<identity>
<dns value="localhost" />
</identity>
</endpoint>
</service>
</services>
</system.serviceModel>
6. Add a .xml file to the project named crossdomain.xml and add the below entries.
<?xml version="1.0"?>
<!DOCTYPE cross-domain-policy SYSTEM "http://www.macromedia.com/xml/dtds/cross-domain-policy.dtd">
<cross-domain-policy>
<allow-http-request-headers-from domain="*" headers="*"/>
</cross-domain-policy>
7. Build the project.
8. Now, lets move to the SilverLight Project. When you add the Silverlight project, it would by default add App.xaml, Page.xaml files. So, at this point of time, we will live with them.
9. Right click on References, and choose Add Service Reference.
10. When the Add Service Reference pop's up... Click on Discover and that should display the SPConnect Service that we added to the solution.
11. Give a name to the namespance '... some thing like SharePointConnect.
12. Get a feel of the object browser and look whats in SharePointConnect.
13. Most importantly you would want to concentrate on the methods / events
a. GetSPListItemsCompleted
b. GetSPListItemsAsync
c. GetSPListItemsCompleted
14. The Page.xaml would look like this.
<UserControl x:Class="LearnSL.Page"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:Controls="clr-namespace:System.Windows.Controls;assembly=System.Windows.Controls.Data"
Width="800" Height="600">
<Grid x:Name="LayoutRoot" Background="White">
<Grid.RowDefinitions>
<RowDefinition Height="10" />
<RowDefinition Height="50" />
<RowDefinition Height="*" />
<RowDefinition Height="10" />
</Grid.RowDefinitions>
<Grid.ColumnDefinitions>
<ColumnDefinition Width="10"/>
<ColumnDefinition Width="*"/>
<ColumnDefinition Width="10"/>
</Grid.ColumnDefinitions>
<Border BorderBrush="Black" BorderThickness="2" Grid.Row="1" Grid.Column="1" />
<StackPanel Grid.Row="1" Grid.Column="1" Orientation="Horizontal">
</StackPanel>
<Controls:DataGrid x:Name="theGrid" AlternatingRowBackground="Beige" AutoGenerateColumns="True"
Width="800" Height="600" Grid.Row="2" Grid.Column="1" CanUserResizeColumns="True">
</Controls:DataGrid>
</Grid>
</UserControl>
15. And the Page.xaml.cs looks like below
using System;
using System.Windows;
using System.Windows.Controls;
namespace LearnSL
{
public partial class Page : UserControl
{
public Page()
{
InitializeComponent();
Loaded += new RoutedEventHandler(Page_Loaded);
}
void Page_Loaded(object sender, RoutedEventArgs e)
{
GetSPList("http://localhost:12345");
}
void GetSPList(string Url)
{
SharePointConnect.SPConnectClient oSPConnectClient = new SharePointConnect.SPConnectClient();
oSPConnectClient.GetSPListItemsCompleted += new EventHandler(oSPConnectClient_GetSPListItemsCompleted);
oSPConnectClient.GetSPListItemsAsync(Url);
}
void oSPConnectClient_GetSPListItemsCompleted(object sender, LearnSL.SharePointConnect.GetSPListItemsCompletedEventArgs e)
{
theGrid.ItemsSource = e.Result;
}
}
}
16. And finally, the ServiceReferences.ClientConfig file would have the below code
<configuration>
<system.serviceModel>
<bindings>
<basicHttpBinding>
<binding name="BasicHttpBinding_ISPConnect" maxBufferSize="2147483647"
maxReceivedMessageSize="2147483647">
<security mode="None" />
</binding>
</basicHttpBinding>
</bindings>
<client>
<endpoint address="http://localhost:11284/SPConnect.svc" binding="basicHttpBinding"
bindingConfiguration="BasicHttpBinding_ISPConnect" contract="SharePointConnect.ISPConnect"
name="BasicHttpBinding_ISPConnect" />
</client>
</system.serviceModel>
</configuration>
17. Now, build the solution and run the Silverlight project and see the output for your self.
In part II I would add some more stuff like how to get specific fields from a SPListt and display in Silverlight grid.
Till then - Have Fun.
Comments